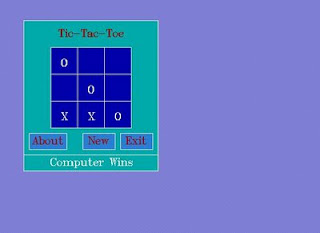
This is a famous game. Here it is written in Turbo c. In this game one player is the user and the other is the computer.
The important feature in this is that the user can use the mouse to select the cells. I wrote this program mainly to introduce how to use mouse in your c programs.
1) For using mouse you must declare the header file ‘bios.h’.
2) Another thing is a union should be declared as follows:
union REGS regs;
3) The mouse can be initialized as follows
regs.x.ax=0;
int86(0x33,®s,®s);
4) To show the mouse
regs.x.ax=1;
int86(0x33,®s,®s);
5) To hide the mouse
regs.x.ax=2;
int86(0x33,®s,®s);
6) To read the co ordinate (position)
regs.x.ax=3;
int86(0x33,®s,®s);
left = regs.x.bx&1; //to get left click
right = regs.x.bx&2;// right click
x = regs.x.cx; // x –coordinate
y= regs.x.dx; // y –coordinate
Here left, right, x, y is in integer data type.
These are the main instructions use full for writing mouse using programs. If the graphics is initialized in your program then the mouse pointer will have an arrow head. Otherwise it will be a rectangular block.
The following strategy can be used while writing these programs.
Coordinate ()
{
Readmouse();
If(x>50 && x<150>25 &&y<50)
Click(1);
...
}
Click(int z)
{
Readmouse();
While(left)
{
Readmouse();
Switch(z)
{
…
}
}
}
Download and Enjoy the game.Tic-Tac- Toe :- Program
/* Tic Tac Toe - Game
* Vipin p nair *
* 25-5-09 *
* College of Engineering Kallooppara *
* vipinpn@gmail.com *
* Kerala *
*/
#include "graphics.h"
#include "bios.h"
#include "conio.h"
#include "stdlib.h"
#include "time.h"
#define CELL1 (x_cord>100 && x_cord<150>100 && y_cord<150)
#define CELL2 (x_cord>150 && x_cord<200>100 && y_cord<150)
#define CELL3 (x_cord>200 && x_cord<250>100 && y_cord<150)
#define CELL4 (x_cord>100 && x_cord<150>150 && y_cord<200)
#define CELL5 (x_cord>150 && x_cord<200>150 && y_cord<200)
#define CELL6 (x_cord>200 && x_cord<250>150 && y_cord<200)
#define CELL7 (x_cord>100 && x_cord<150>200 && y_cord<250)
#define CELL8 (x_cord>150 && x_cord<200>200 && y_cord<250)
#define CELL9 (x_cord>200 && x_cord<250>200 && y_cord<250)
#define EXIT (x_cord>230 && x_cord<290>260 && y_cord<290)
#define NEW (x_cord>160 && x_cord<220>260 && y_cord<290)
#define ABOUT (x_cord>60 && x_cord<130>260 && y_cord<290)
void PlyrComp();
union REGS regs;
int left_click,
x_cord,
y_cord,
select[9],
selectcmp[9];
char pass,pass1,pass2,win=1;
// mouse functions
void InitMouse()
{
// initialize
regs.x.ax=0;
int86(0x33,®s,®s);
}
void showmouse()
{
// show mouse
regs.x.ax=1;
int86(0x33,®s,®s);
}
void hidemouse()
{
regs.x.ax=2;
int86(0x33,®s,®s);
}
void readmouse()
{
regs.x.ax = 3;
int86(0x33,®s,®s);
left_click = regs.x.bx & 1;
// right_click = regs.x.bx & 2
x_cord = regs.x.cx;
y_cord = regs.x.dx;
}
// graphics functions
void InitGraphics()
{
int gdriver=DETECT,gmode,errorcode;
initgraph(&gdriver,&gmode,"");
/* read result of initialization */
errorcode = graphresult();
if (errorcode != grOk) /* an error occurred */
{
printf("Graphics error: %s\n", grapherrormsg(errorcode));
printf("Press any key to halt:");
getch();
exit(1); /* terminate with an error code */
}
}
void MainScreen()
{
setbkcolor(CYAN);
setcolor(WHITE);
rectangle(100,100,250,250);
setfillstyle(1,BLUE);
floodfill(150,150,WHITE);
line(100,150,250,150);
line(100,200,250,200);
line(150,100,150,250);
line(200,100,200,250);
rectangle(50,50,300,300);
rectangle(230,260,290,290);
rectangle(160,260,220,290);
rectangle(50,300,300,330);
rectangle(60,260,130,290);
setfillstyle(9,LIGHTBLUE);
floodfill(240,270,WHITE);
floodfill(170,270,WHITE);
floodfill(70,270,WHITE);
setfillstyle(9,LIGHTMAGENTA);
floodfill(350,350,WHITE);
settextstyle(1,0,2);
setcolor(RED);
outtextxy(115,60,"Tic-Tac-Toe");
outtextxy(240,260,"Exit");
outtextxy(170,260,"New");
outtextxy(66,260,"About");
setcolor(WHITE);
select[0]=0;select[1]=0;select[2]=0;
select[3]=0;select[4]=0;select[5]=0;
select[6]=0;select[7]=0;select[8]=0;
selectcmp[0]=0;selectcmp[1]=0;selectcmp[2]=0;
selectcmp[3]=0;selectcmp[4]=0;selectcmp[5]=0;
selectcmp[6]=0;selectcmp[7]=0;selectcmp[8]=0;
pass=0;
pass2=0;
PlyrComp();
}
//*************************************************************
void Comptr_click(int a)
{
switch(a)
{
case 0:
case 1: outtextxy(120,115,"O");selectcmp[0]=1;
break;
case 2: outtextxy(170,115,"O");selectcmp[1]=1;
break;
case 3: outtextxy(220,115,"O");selectcmp[2]=1;
break;
case 4: outtextxy(120,165,"O");selectcmp[3]=1;
break;
case 5: outtextxy(170,165,"O");selectcmp[4]=1;
break;
case 6: outtextxy(220,165,"O");selectcmp[5]=1;
break;
case 7: outtextxy(120,215,"O");selectcmp[6]=1;
break;
case 8: outtextxy(170,215,"O");selectcmp[7]=1;
break;
case 9: outtextxy(220,215,"O");selectcmp[8]=1;
break;
}
pass1=1;
}
//**************************************************************
void PlyrComp()
{
int x=0;
// getch();
if(!pass)
{
randomize();
Comptr_click(rand()%10);
pass=1;
return;
}
// final step for computer win
// horiz check
pass1=0;
if ((selectcmp[0]+selectcmp[1]+selectcmp[2])==2)
{
if (((selectcmp[0]+selectcmp[1])==2) && !select[2])
Comptr_click(3);
if (((selectcmp[0]+selectcmp[2])==2) && !select[1])
Comptr_click(2);
if (((selectcmp[1]+selectcmp[2])==2) && !select[0])
Comptr_click(1);
}
if(pass1)return;
pass1=0;
if ((selectcmp[3]+selectcmp[4]+selectcmp[5])==2)
{
if (((selectcmp[3]+selectcmp[4])==2) && !select[5])
Comptr_click(6);
if (((selectcmp[3]+selectcmp[5])==2) && !select[4])
Comptr_click(5);
if (((selectcmp[4]+selectcmp[5])==2) && !select[3])
Comptr_click(4);
}
if(pass1)return;
pass1=0;
if ((selectcmp[6]+selectcmp[7]+selectcmp[8])==2)
{
if (((selectcmp[6]+selectcmp[7])==2) && !select[8])
Comptr_click(9);
if (((selectcmp[6]+selectcmp[8])==2) && !select[7])
Comptr_click(8);
if (((selectcmp[7]+selectcmp[8])==2) && !select[6])
Comptr_click(7);
}
if(pass1)return;
// vertical check
pass1=0;
if ((selectcmp[0]+selectcmp[3]+selectcmp[6])==2)
{
if (((selectcmp[0]+selectcmp[3])==2) && !select[6])
Comptr_click(7);
if (((selectcmp[0]+selectcmp[6])==2) && !select[3])
Comptr_click(4);
if (((selectcmp[3]+selectcmp[6])==2) && !select[0])
Comptr_click(1);
}
if(pass1)return;
pass1=0;
if ((selectcmp[1]+selectcmp[4]+selectcmp[7])==2)
{
if (((selectcmp[1]+selectcmp[4])==2) && !select[7])
Comptr_click(8);
if (((selectcmp[1]+selectcmp[7])==2) && !select[4])
Comptr_click(5);
if (((selectcmp[4]+selectcmp[7])==2) && !select[1])
Comptr_click(2);
}
if(pass1)return;
pass1=0;
if ((selectcmp[2]+selectcmp[5]+selectcmp[8])==2)
{
if (((selectcmp[2]+selectcmp[5])==2) && !select[8])
Comptr_click(9);
if (((selectcmp[2]+selectcmp[8])==2) && !select[5])
Comptr_click(6);
if (((selectcmp[5]+selectcmp[8])==2) && !select[2])
Comptr_click(3);
}
if(pass1)return;
pass1=0;
if ((selectcmp[0]+selectcmp[4]+selectcmp[8])==2)
{
if (((selectcmp[0]+selectcmp[4])==2) && !select[8])
Comptr_click(9);
if (((selectcmp[0]+selectcmp[8])==2) && !select[4])
Comptr_click(5);
if (((selectcmp[8]+selectcmp[4])==2) && !select[0])
Comptr_click(1);
}
if(pass1)return;
pass1=0;
if ((selectcmp[2]+selectcmp[4]+selectcmp[6])==2)
{
if (((selectcmp[2]+selectcmp[4])==2) && !select[6])
Comptr_click(7);
if (((selectcmp[2]+selectcmp[6])==2) && !select[4])
Comptr_click(5);
if (((selectcmp[6]+selectcmp[4])==2) && !select[2])
Comptr_click(3);
}
if(pass1)return;
// blocking plyr winning conditions
// horiz check
pass1=0;
if ((select[0]+select[1]+select[2])==2)
{
if (((select[0]+select[1])==2) && !selectcmp[2])
Comptr_click(2+1);
if (((select[0]+select[2])==2) && !selectcmp[1])
Comptr_click(1+1);
if (((select[1]+select[2])==2) && !selectcmp[0])
Comptr_click(0+1);
}
if(pass1)return;
pass1=0;
if ((select[3]+select[4]+select[5])==2)
{
if (((select[3]+select[4])==2) && !selectcmp[5])
Comptr_click(5+1);
if (((select[3]+select[5])==2) && !selectcmp[4])
Comptr_click(4+1);
if (((select[4]+select[5])==2) && !selectcmp[3])
Comptr_click(3+1);
}
if(pass1)return;
pass1=0;
if ((select[6]+select[7]+select[8])==2)
{
if (((select[6]+select[7])==2) && !selectcmp[8])
Comptr_click(8+1);
if (((select[6]+select[8])==2) && !selectcmp[7])
Comptr_click(7+1);
if (((select[7]+select[8])==2) && !selectcmp[6])
Comptr_click(6+1);
}
if(pass1)return;
// vertical check
pass1=0;
if ((select[0]+select[3]+select[6])==2)
{
if (((select[0]+select[3])==2) && !selectcmp[6])
Comptr_click(6+1);
if (((select[0]+select[6])==2) && !selectcmp[3])
Comptr_click(3+1);
if (((select[3]+select[6])==2) && !selectcmp[0])
Comptr_click(0+1);
}
if(pass1)return;
pass1=0;
if ((select[1]+select[4]+select[7])==2)
{
if (((select[1]+select[4])==2) && !selectcmp[7])
Comptr_click(7+1);
if (((select[1]+select[7])==2) && !selectcmp[4])
Comptr_click(4+1);
if (((select[4]+select[7])==2) && !selectcmp[1])
Comptr_click(1+1);
}
if(pass1)return;
pass1=0;
if ((select[2]+select[5]+select[8])==2)
{
if (((select[2]+select[5])==2) && !selectcmp[8])
Comptr_click(8+1);
if (((select[2]+select[8])==2) && !selectcmp[5])
Comptr_click(5+1);
if (((select[5]+select[8])==2) && !selectcmp[2])
Comptr_click(2+1);
}
if(pass1)return;
pass1=0;
if ((select[0]+select[4]+select[8])==2)
{
if (((select[0]+select[4])==2) && !selectcmp[8])
Comptr_click(8+1);
if (((select[0]+select[8])==2) && !selectcmp[4])
Comptr_click(4+1);
if (((select[8]+select[4])==2) && !selectcmp[0])
Comptr_click(0+1);
}
if(pass1)return;
pass1=0;
if ((select[2]+select[4]+select[6])==2)
{
if (((select[2]+select[4])==2) && !selectcmp[6])
Comptr_click(6+1);
if (((select[2]+select[6])==2) && !selectcmp[4])
Comptr_click(4+1);
if (((select[6]+select[4])==2) && !selectcmp[2])
Comptr_click(2+1);
}
if(pass1)return;
pass1=0;
while(pass2 && (checkwin()==3) && !pass1)
{
x=rand()%10;
if( (!select[x-1]) && (!selectcmp[x-1]) )
{
Comptr_click(x);
return;
}
}
}
//****************************************************************
int checkwin()
{
int i;
// return 1 for player win , 2 for computer & 0 for draw
// horiz
if (select[0] && select[1] && select[2]) return 1;
if (select[3] && select[4] && select[5]) return 1;
if (select[6] && select[7] && select[8]) return 1;
// vert
if (select[0] && select[3] && select[6]) return 1;
if (select[1] && select[4] && select[7]) return 1;
if (select[2] && select[5] && select[8]) return 1;
// diag
if (select[0] && select[4] && select[8]) return 1;
if (select[6] && select[4] && select[2]) return 1;
// comp
// horiz
if (selectcmp[0] && selectcmp[1] && selectcmp[2]) return 2;
if (selectcmp[3] && selectcmp[4] && selectcmp[5]) return 2;
if (selectcmp[6] && selectcmp[7] && selectcmp[8]) return 2;
// vert
if (selectcmp[0] && selectcmp[3] && selectcmp[6]) return 2;
if (selectcmp[1] && selectcmp[4] && selectcmp[7]) return 2;
if (selectcmp[2] && selectcmp[5] && selectcmp[8]) return 2;
// diag
if (selectcmp[0] && selectcmp[4] && selectcmp[8]) return 2;
if (selectcmp[6] && selectcmp[4] && selectcmp[2]) return 2;
for (i=0;i<9;i++)
if(!(select[i]||selectcmp[i]))
return 3;
return 0;
}
//****************************************************************8
int check(int a)
{
if (a==9 || a==10) return 0;
if (select[a] || selectcmp[a])
return 1;
return 0;
}
//****************************************************************
result()
{
switch(checkwin())
{
case 1: outtextxy(100,300,"Player Wins");return 0;
case 2: outtextxy(100,300,"Computer Wins"); return 0;
case 0: outtextxy(100,300,"Game Draw"); return 0;
}
}
//******************************************************************
void click(int z)
{
readmouse();
while(left_click)
{
left_click=0;
// readmouse();
if(check(z-1))return;
if (z<10)
pass2=z;
else pass2=0;
hidemouse();
switch(z)
{
case 1: outtextxy(120,115,"X");select[0]=1;
break;
case 2: outtextxy(170,115,"X");select[1]=1;
break;
case 3: outtextxy(220,115,"X");select[2]=1;
break;
case 4: outtextxy(120,165,"X");select[3]=1;
break;
case 5: outtextxy(170,165,"X");select[4]=1;
break;
case 6: outtextxy(220,165,"X");select[5]=1;
break;
case 7: outtextxy(120,215,"X");select[6]=1;
break;
case 8: outtextxy(170,215,"X");select[7]=1;
break;
case 9: outtextxy(220,215,"X");select[8]=1;
break;
case 10: cleardevice();
exit(0);
case 11: cleardevice();
MainScreen();
pass=0;
break;
case 12: outtextxy(70,330,"Vipin P Nair");
outtextxy(70,360,"College Of Engineering Kallooppara") ;
outtextxy(70,390,"Kerala, India");
break;
}
// delay(450);
showmouse();
result();
PlyrComp();
win=result();
}
}
//****************************************************************
void cordinate()
{
readmouse();
if (win)
{
if(CELL1)click(1);
if(CELL2)click(2);
if(CELL3)click(3);
if(CELL4)click(4);
if(CELL5)click(5);
if(CELL6)click(6);
if(CELL7)click(7);
if(CELL8)click(8);
if(CELL9)click(9);
}
if(EXIT) click(10);
if(NEW) click(11);
if(ABOUT)click(12);
}
//**************************************************************
void main()
{
InitGraphics();
MainScreen();
InitMouse();
showmouse();
while(1)
cordinate();
}
No comments:
Post a Comment